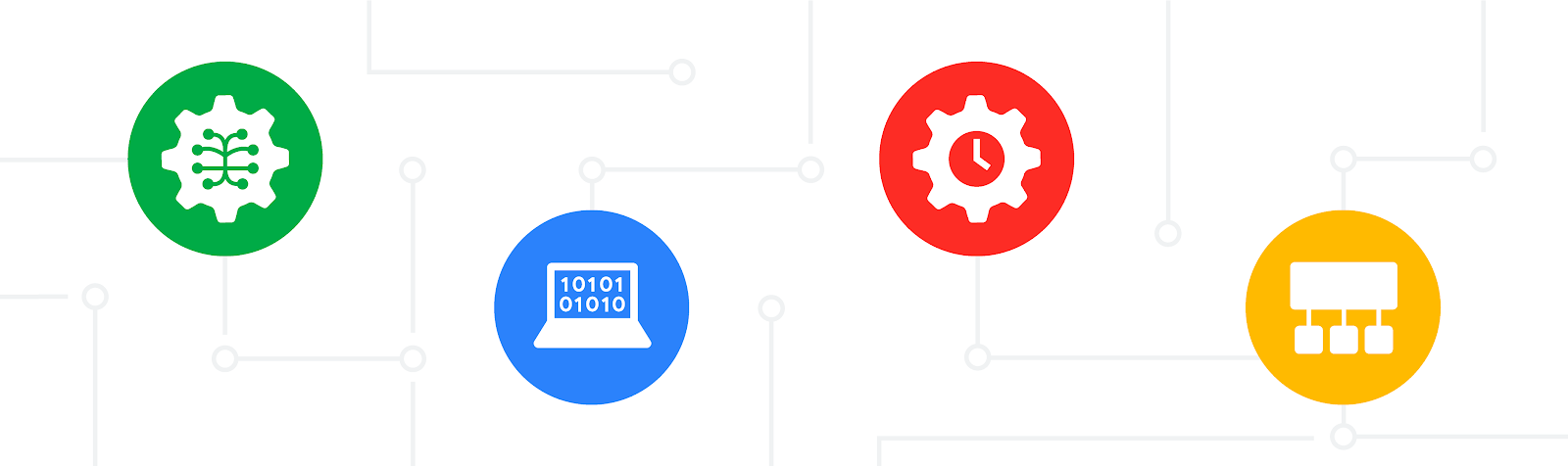
Buffer overflows are the cause of many security issues, and are a persistent thorn in programmers' sides. C is particularly susceptible to them. The advent of sanitizers mitigates some security issues by automatically inserting bounds checking, but they're not able to do so in all situations—in particular for flexible array members, because their size is known only at runtime.
The size of a flexible array member is typically opaque to the compiler. The alloc_size attribute on malloc() may be used for bounds checking flexible array members within the same function as the allocation. But the attribute's information isn't carried with the allocated object, making it impossible to perform bounds checking elsewhere.
To mitigate this drawback, Clang and GCC are introducing1 the counted_by attribute for flexible array members.
Specifying a flexible array member's element count
The number of elements allocated for a flexible array member is frequently stored in another field within the same structure. When applied to the flexible array member, the counted_by attribute is used by the sanitizer—enabled by -fsanitize=array-bounds—by explicitly referencing the field that stores the number of elements. The attribute creates an implicit relationship between the flexible array member and the count field enabling the array bounds sanitizer to verify flexible array operations.
There are some rules to follow when using this feature. For this structure:
struct foo {
/* ... */
size_t count; /* Number of elements in array */
int array[] __attribute__((counted_by(count)));
};
- The count field must be within the same non-anonymous, enclosing struct as the flexible array member.
- The count field must be set before any array access.
- The array field must have at least count number of elements available at all times.
- The count field may change, but must never be larger than the number of elements originally allocated.
An example allocation of the above structure:
struct foo *foo_alloc(size_t count) {
struct foo *ptr = NULL;
size_t size = MAX(sizeof(struct foo),
offsetof(struct foo, array[0]) +
count * sizeof(p->array[0]));
ptr = calloc(1, size);
ptr->count = count;
return ptr;
}
Uses for fortification
Fortification (enabled by the _FORTIFY_SOURCE macro) is an ongoing project to make the Linux kernel more secure. Its main focus is preventing buffer overflows on memory and string operations.
Fortification uses the __builtin_object_size() and __builtin_dynamic_object_size() builtins to try to determine if input passed into a function is valid (i.e. "safe"). A call to __builtin_dynamic_object_size() generally isn't able to take the size of a flexible array member into account. But with the counted_by attribute, we're able to calculate the size and improve safety.
Uses in the Linux kernel
The counted_by attribute is already in use in the Linux kernel, and will be instrumental in catching issues like integer overflows, which led to a heap buffer overflow. We want to expand its use to more flexible array members, and enforce its use in the future.
Conclusion
The counted_by attribute helps address a long-standing fortification road block where the memory bounds of a flexible array member couldn't be determined by the compiler, thus making Linux, and other hardened applications, less exploitable.
1In Clang v18.0.0 and GCC v15.0.0.
By Bill Wendling, Staff Software Engineer